Hello good day! We're currently working on a project. To try the functions that we'll be using we used this repo for the arduino code
We're trying to read a string from a textbox in MIT app. However, it seems that the blocks or something with the code aren't correct. We're using SIM800L and HC-05 Bluetooth modules. The SIM800L is working fine if we're to input the number directly into the code. I would like to ask for help or corrections that might be affecting the code. We're using Arduino UNO
Here's our designer and blocks
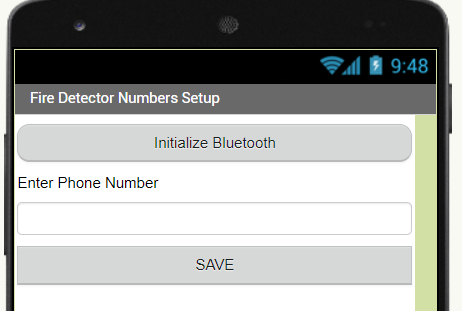
Here's the code that we're testing
#include <SoftwareSerial.h>
//--------PHONE NUMBERS------------------
String PHONE_1 = "";
//---------COMPONENT PINS----------------
#define buzzer_pin 4
#define flame_sensor_pin 5
#define button_pin 6
//---------SIM800L PINS------------------
#define rxPin 2
#define txPin 3
//--------BLUETOOTH PINS------------------
#define bTrxPin 0
#define bTtxPin 1
//-------SERIAL--------------------------
SoftwareSerial sim800L(rxPin, txPin);
SoftwareSerial BTSerial(bTrxPin, bTtxPin);
//---------if 0 - no fire----------------
//---------if 1 - there's fire-----------
boolean fire_flag = 0;
//----------VOID SETUP-------------------
void setup() {
//Begin serial communication for Arduino
Serial.begin(115200);
//Begin serial communication for bluetooth
BTSerial.begin(9600);
//Begin serial communication for bluetooth
pinMode(bTrxPin, INPUT);
pinMode(bTtxPin, OUTPUT);
BTSerial.begin(9600);
//---------PINMODE SETUP----------------
pinMode(flame_sensor_pin, INPUT);
pinMode(button_pin, INPUT);
pinMode(buzzer_pin, OUTPUT);
digitalWrite(buzzer_pin, LOW);
//---------INITIALIZE SIM800L-----------
Serial.println("Initializing...");
//Once the handshake test is successful, it will back to OK
sim800L.println("AT");
delay(1000);
sim800L.println("AT+CMGF=1");
delay(1000);
}
void loop(){
int flame_value = digitalRead(flame_sensor_pin);
int buttonState = digitalRead(button_pin);
while(sim800L.available()){
Serial.println(sim800L.readString());
}
while(BTSerial.available()>0){
PHONE_1 = BTSerial.readString();
//fire on
if(flame_value == LOW){
digitalWrite(buzzer_pin, HIGH);
if(fire_flag == 0)
{
Serial.println("Fire Detected.");
fire_flag == 1;
send_multi_sms();
make_multi_call();
}
}
else if(buttonState == HIGH){
digitalWrite(buzzer_pin, HIGH);
if(fire_flag == 0)
{
Serial.println("Button is Pushed! Someone detected fire!");
fire_flag == 1;
send_multi_sms();
make_multi_call();
}
}
//fire OFF
else{
digitalWrite(buzzer_pin,LOW);
fire_flag = 0;
}
}
//fire ON
}
//-----------SEND SMS-----------
void send_multi_sms()
{
if(PHONE_1 != ""){
Serial.print("Phone 1: ");
send_sms("Fire is Detected amorlina liwat nori", PHONE_1);
}
}
//-----------MAKE CALL-----------
void make_multi_call()
{
if(PHONE_1 != ""){
Serial.print("Phone 1: ");
make_call(PHONE_1);
}
}
//--------------MESSAGE---------------------------------
void send_sms(String text, String phone)
{
Serial.println("sending sms....");
delay(50);
sim800L.print("AT+CMGF=1\r");
delay(1000);
sim800L.print("AT+CMGS=""+phone+""\r");
delay(1000);
sim800L.print(text);
delay(100);
sim800L.write(0x1A); //ascii code for ctrl-26 //Serial2.println((char)26); //ascii code for ctrl-26
delay(5000);
}
//-------------CALLING----------------------------------
void make_call(String phone)
{
Serial.println("calling....");
sim800L.println("ATD"+phone+";");
delay(20000); //20 sec delay
sim800L.println("ATH");
delay(1000); //1 sec delay
}