Hello friends,
To upload and download Firebase storage files we can use several methods, here are some:
I have also used JavaScript in my tutorial in Spanish and I will share it in this topic.
0.- Configuration. Rules. Settings. Add app Web.
- For testing I will use the public rules
rules_version = '2';
service firebase.storage {
match /b/{bucket}/o {
match /{allPaths=**} {
allow read, write;
}
}
}
rules_version = '2' is necessary for the "List files" code.
-
Settings.
-
Add app / web app.
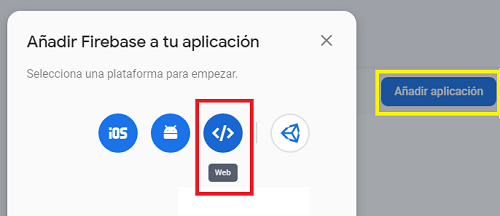
1.- Web page JavaScript.
- Copy & Paste this firebasestorage.htm code in a file in your PC. Change firebaseConfig and run with a Browser.
- You can Upload file (or several), get url file, get list files of Firebase storage.
<!DOCTYPE html>
<html><head>
<meta name="viewport" content="width=device-width, initial-scale=4, maximum-scale=1" />
</head><body>
<div>
<input type="file" id="files" multiple /><br /><br />
<button id="send">Upload</button>
<p id="uploading"></p>
<progress value="0" max="100" id="progress"></progress>
<br /><br /><br /><br />
<input type="text" name="nombre" id="name" value ="hello.wav" /><br /><br />
<button id="get_url">Get url file</button>
<p id="response"></p>
<button id="list_files">List files</button>
<p id="response_list"></p>
</div>
<script src="https://www.gstatic.com/firebasejs/7.15.1/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.15.1/firebase-storage.js"></script>
<script>
var firebaseConfig = {
apiKey: "AIzaSyADXXXXXXXXXXXXXXXXXXXXXXXXX-fEk",
authDomain: "kio4.firebaseapp.com",
databaseURL: "https://kio4.firebaseio.com",
projectId: "firebase-kio4",
storageBucket: "firebase-kio4.appspot.com",
// messagingSenderId: "851XXXXXXXX7",
// appId: "1:851XXXXXXXX7:web:6fXXXXXXXXXX866"
};
firebase.initializeApp(firebaseConfig);
</script>
<script>
//// CHOICE /////
var files = [];
document.getElementById("files").addEventListener("change", function(e) {
files = e.target.files;
});
///// SEND FILES //////
document.getElementById("send").addEventListener("click", function() {
if (files.length != 0) {
for (let i = 0; i < files.length; i++) {
var storage = firebase.storage().ref(files[i].name);
var upload = storage.put(files[i]);
upload.on("state_changed", function progress(snapshot) {
var percentage = (snapshot.bytesTransferred / snapshot.totalBytes) * 100;
document.getElementById("progress").value = percentage;
},
function error() {alert("Error uploading file.");},
function complete() {document.getElementById( "uploading").innerHTML += `${files[i].name} uploaded <br />`;}); }
} else {alert("No file chosen."); }
});
////////////////////////////////////
// GET URL ////////////////////
document.getElementById("get_url").addEventListener("click", function() {
var get_url_file = document.getElementById("name").value;
var storage = firebase.storage().ref(get_url_file);
storage.getDownloadURL().then(function(url) {document.getElementById("response").innerHTML += `${url} <br />`; })
.catch(function(error) {alert("Error encountered."); });
});
////////////////////////////////////
// LIST FILES ////////////////////
document.getElementById("list_files").addEventListener("click", function() {
var storageRef = firebase.storage().ref();
storageRef.listAll().then(function(result) {
result.items.forEach(function(urlFile) {document.getElementById("response_list").innerHTML += `${urlFile} <br />`; });
}).catch(function(error) {alert("No file chosen."); });
});
////////////////////////////////////
</script>
</body>
</html>
2.- App to get file url and download file with Web component.
p150_firebase_js.aia (3.1 KB)
EDIT: change file:///mnt/sdacard/AppInventor/assets/fbs_geturl.htm
by
http://localhost/fbs_geturl.htm
<!DOCTYPE html>
<html><head></head><body>
<script src="https://www.gstatic.com/firebasejs/7.15.1/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.15.1/firebase-storage.js"></script>
<script>
var firebaseConfig = {
apiKey: "AIzaSyADXXXXXXXXXXXXXXXXXXXXXXXXX-fEk",
authDomain: "kio4.firebaseapp.com",
databaseURL: "https://kio4.firebaseio.com",
projectId: "firebase-kio4",
storageBucket: "firebase-kio4.appspot.com",
// messagingSenderId: "851XXXXXXXX7",
// appId: "1:851XXXXXXXX7:web:6fXXXXXXXXXX866"
};
firebase.initializeApp(firebaseConfig);
</script>
<script>
datos = window.AppInventor.getWebViewString(); // Input.
var get_url_file = datos;
var storage = firebase.storage().ref(get_url_file);
storage.getDownloadURL().then(function(url) { window.AppInventor.setWebViewString("" + url); }) // Output.
.catch(function(error) {alert("Error encountered."); });
</script>
</body>
</html>
oooooooooooooooooooooooo00000ooooooooooooooooooo
To delete file:
storage.delete().then(function(url) { window.AppInventor.setWebViewString("Deleted."); }) // Output.
.catch(function(error) {alert("Error encountered."); });
3.- Upload file.
p150_firebase_js_2.aia (5.3 KB)
- We cannot choose files and upload them directly with the WebViewer component, so we will open the browser that has the default device and open the page fbs_geturl.htm
4.- Upload File by Web component.
p150_firebase_js_3.aia (6.2 KB)
https://firebasestorage.googleapis.com/v0/b/[storageBucket]/o/namefile.abc
(We assume public rules, read, write.)
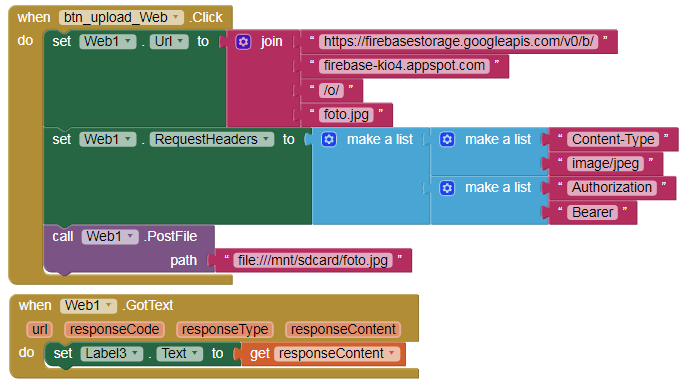
- In Content-Type:
image/gif, image/png, image/jpeg, image/bmp, image/webp, audio/midi, audio/mpeg, audio/webm, audio/ogg, audio/wav, application/octet-stream, application/pkcs12, application/vnd.mspowerpoint, application/xhtml+xml, application/xml, application/pdf, MIME Office.
5.- Save files to FirebaseDB or CloudDB with Base 64 extension.
Although Database / Realtime is mainly intended to save short data, we can also use it to save files by previously converting them to Base 64 using an extension.
1 Like
Muchas gracias, Juan Antonio, por el esfuerzo que has puesto en hacer "la Biblia" de como subir y bajar archivos a Firebase Storage.
Al final encontré una forma sencilla de bajar archivos desde Storage al almacenamiento local del móvil, con 4 bloques de AppInventor:
1 Like
One more block could be required, in order to reset the RequestHeaders, if you want to download a file (with .Get) after previously having uploaded a file (with .PostFile).
Or else, you might get an error: SignatureDoesNotMatch

6.- Create QR image, upload it to FireBase Storage by Web component. Gets the url of the image.
p150i_firebase_js_4.aia (4.9 KB)
-
I have used Android 9, for Android 10+ you will have to change the directory of the SdCard.
-
Write a text (Name, address, city, age) and create a QR image file using:
https://quickchart.io/documentation/#qr
-
Load that image into an ImageSprite. Save that Canvas as image on the SdCard.
-
Upload the image to FireBase Storage using the Web component.
-
Through responseContent it obtains the url of the image.
7.- Upload and Download file with FirebaseStorage extension.
p150_firebase_6.aia (54.2 KB)
8.- Download Image and install file, from Firebase storage.
Using the "Upload file" button we upload an image file and .apk installer file to Firebase.
Now we are going to download them through an application.
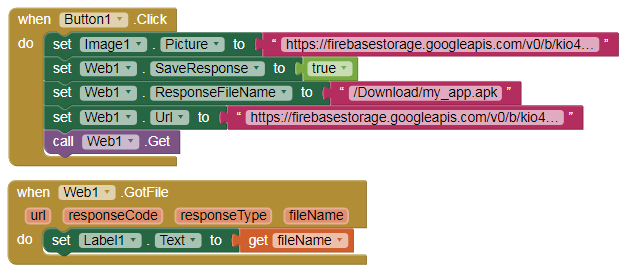
- We establish the links of the two files.
- The image is inserted into an Image component.
- The .apk file is saved in the directory
/storage/emulated/0/Download/my_app.apk [Only tested on Android 9]
- DefaultFileScope = Legacy
p150_firebase_7.aia (35.9 KB)
1 Like