In this tutorial we saw how we could communicate ESP32, Firebase realtime and an application...
https://community.appinventor.mit.edu/t/esp32-firebasedb-send-receive-ide-arduino/10747
We are going to adapt those codes to ESP8266.
- We can send data from the App to Firebase and display it in the Serial Monitor.
- The ESP8266 code generates two random numbers every 5 seconds, sends them to Firebase and is automatically received by the app.
We will use this library...
We will adapt this code...
https://github.com/mobizt/Firebase-ESP8266/blob/master/examples/Basic/Basic.ino
We will need the address of our project in Firebase realtime...
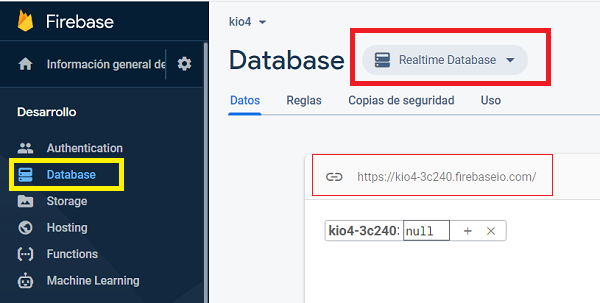
p117_wemos_firebase_ESP8266.aia (2.9 KB)
We set that FirebaseURL in the Designer.
We will also put a ProjectBucket, in this example ESP8266
When the DataChanged in Firebase, we will get its values.
We can also send data to Firebase.
ESP8266_Firebase.ino
// Juan A. Villalpando
// http://kio4.com/arduino/117_ESP8266_Firebase.htm
#include <ESP8266Firebase.h>
#include <ESP8266WiFi.h>
// SLOW BUT HASTLE-FREE METHOD FOR LONG TERM USAGE.
// DOES NOT REQUIRE PERIODIC UPDATE OF FINGERPRINT
Firebase firebase("kio4b-3C240");
const char* ssid = "Nombre_Red_WiFi";
const char* password = "Clave_WiFi";
float temperatura = 25.0;
float humedad = 87.0;
String texto = "Hola";
String numero = "123";
unsigned long tiempo_actual = 0;
void setup() {
Serial.begin(115200);
delay(10);
Serial.println();
Serial.print("Connecting with ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("WiFi conected. IP: ");
Serial.println(WiFi.localIP());
}
void loop() {
// RECEIVE from App
if (firebase.getString("ESP8266/numero")) {
String numero_fb = firebase.getString("ESP8266/numero");
if (numero_fb != numero) {
numero = numero_fb;
Serial.println(numero);
}
}
if (firebase.getString("ESP8266/texto")) {
String texto_fb = firebase.getString("ESP8266/texto");
if (texto_fb != texto) {
texto = texto_fb;
Serial.println(texto);
}
}
///////////////////////////////////////
// SEND to App 2 random numbers every 5 seconds.
if((millis()- tiempo_actual)>= 5000){
temperatura = random(0,10000)/100.0;
humedad = random(0,10000)/100.0;
String datos = (String) temperatura + "|" + (String) humedad;
Serial.println(datos);
//firebase.setFloat("ESP8266/temperatura", temperatura);
//firebase.setFloat("ESP8266/humedad", humedad);
firebase.setString("ESP8266/datos", datos);
tiempo_actual = millis();
}
}
Result.
This tutorial in Spanish.
http://kio4.com/arduino/117B_ESP8266_Firebase.htm
2 Likes
- Trying different libraries.
I have been doing tests with different libraries for ESP8266 and Firebase.
1.- Library <ESP8266Firebase.h> by Rapak Poddar
- It only needs the firebase address: Firebase firebase("kio4b-3C240");
- It does not need a secret key or api key.
- It's a bit slow in responses.
- The author says...
// SLOW BUT HASTLE-FREE METHOD FOR LONG TERM USAGE.
// DOES NOT REQUIRE PERIODIC UPDATE OF FINGERPRINT
2.- Library <FirebaseArduino.h>
https://docs.particle.io/reference/device-os/libraries/f/FirebaseArduino/
- It has a quick response.
- It Needs Firebase project address and Firebase secret key.
#define Firebase_direccion "kio4b-60981.firebaseio.com"
#define Firebase_secret "C5PQ6-secret_Firebase_I22U3OpBx"
From time to time you must change the Fingerprint of Firebase in the library/FirebaseArduino/src/FirebaseHttpClient.h file
Read this:
https://github.com/FirebaseExtended/firebase-arduino/issues/236
3.- Library <FirebaseESP8266.h> by mobizt
https://github.com/mobizt/Firebase-ESP8266
- I think it is the best library.
- It has a quick response.
- Works with ESP32 and with ESP8266.
- It Needs Firebase project address and Firebase secret key.
#define DATABASE_URL "kio4b-60981.firebaseio.com"
config.signer.tokens.legacy_token = "C5PQ6Ie_clave_secret_de_Firebase_n5I22U3OpBx";
I have put several examples in...
http://kio4.com/arduino/117B_ESP8266_Firebase.htm
I have a question to 3.- Library <FirebaseESP8266.h> by mobizt**
How to send in string type data, bcs i cant seem to do so.
Firebase.setFloat works perfecty fine,
but Firebase.setString does not.
Can u give me a tip?
I have it like that:
Firebase.setString(firebaseTemperatura,"/project-787465550920/Indukcja/",msg1);
Firebase.setFloat (firebaseTemperatura,"/project-787465550920/Temperatura/",s);
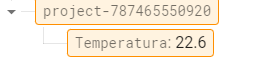
2.- Through the Serial Monitor we send a message as a character string to Firebase.
- I have used the library of mobitz.
#include <Arduino.h>
#if defined(ESP32)
#include <WiFi.h>
#include <FirebaseESP32.h>
#elif defined(ESP8266)
#include <ESP8266WiFi.h>
#include <FirebaseESP8266.h>
#elif defined(PICO_RP2040)
#include <WiFi.h>
#include <FirebaseESP8266.h>
#endif
#define WIFI_SSID "Tu_Red_WiFi"
#define WIFI_PASSWORD "Tu_Clave_WiFi"
#define DATABASE_URL "kio4b-60980.firebaseio.com"
FirebaseData fbdo;
FirebaseAuth auth;
FirebaseConfig config;
void setup(){
Serial.begin(115200);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.print("Connecting to Wi-Fi");
while (WiFi.status() != WL_CONNECTED)
{
Serial.print(".");
delay(300);
}
Serial.println();
Serial.print("Connected with IP: ");
Serial.println(WiFi.localIP());
Serial.println();
config.signer.test_mode = true;
config.database_url = DATABASE_URL;
config.signer.tokens.legacy_token = "C5PQ6Ts_secret_Firebase_I22U3OpBx";
Firebase.begin(&config, &auth);
Firebase.reconnectWiFi(true);
Firebase.setDoubleDigits(5);
}
void loop(){
if (Serial.available() > 0) {
String data = Serial.readStringUntil('\n');
data.trim();
Serial.printf("Set string... %s\n", Firebase.setString(fbdo, F("/ESP8266_2/dato"), data) ? "ok" : fbdo.errorReason().c_str());
Serial.printf("Get string... %s\n", Firebase.getString(fbdo, F("/ESP8266_2/dato")) ? fbdo.to<const char *>() : fbdo.errorReason().c_str());
Serial.println();
}
}
Ok, but i need to use premade massages. How do i do that then?
And for some reason the code u gave me cant compile this line
Serial.printf("Get string... %s\n", Firebase.getString(fbdo, F("/ESP8266_2/dato")) ? fbdo.to<const char *>() : fbdo.errorReason().c_str());
Have you set
FirebaseData fbdo;
?
void loop(){
if (Serial.available() > 0) {
String data = Serial.readStringUntil('\n');
data.trim();
// Serial.printf("Set string... %s\n", Firebase.setString(fbdo, F("/ESP8266_2/dato"), data) ? "ok" : fbdo.errorReason().c_str());
// Serial.printf("Get string... %s\n", Firebase.getString(fbdo, F("/ESP8266_2/dato")) ? fbdo.to<const char *>() : fbdo.errorReason().c_str());
Firebase.setString(fbdo, F("/ESP8266_2/dato"), data);
Firebase.getString(fbdo, F("/ESP8266_2/dato"));
Serial.println();
}
}
Yes, apparently i couldnt make a string variable and needed to have message typed in loop in "xyz" form.
Also i overlooked that "F" in function and now it works!
Thanks very much!