Hello friends,
this topic is about sending data from app to Raspberry Pi and from Raspberry Pi to app, via Bluetooth. It is based on this tutorial in Spanish, take a look.
http://kio4.com/raspberry/31_bluetooth.htm
1.- Resources I have used.
-
Raspberry Pi 3 Model B
-
Raspbian (Buster)
-
Phyton 2
-
Xiaomi Redmi 7 with Android 9
-
To do the tests I use Windows and run VNC (remote Desktop), this way from Windows I can control the configuration of the Raspberry Pi.
-
In this tutorial you can consult some ideas. http://kio4.com/raspberry/index.htm
3 Likes
2.- App sends data to the Raspberry Pi via Bluetooth.
p9B3i_enviar_RaspBerry.aia (3.3 KB)
-
App sends every 600 ms, sequentially 5 bytes contained in a list.
11001100,
00110010,
11011011,
10110110,
00000000
-
Phyton2. Script : bluetooth4.py
import bluetooth
server_sock=bluetooth.BluetoothSocket(bluetooth.RFCOMM)
port = 22
server_sock.bind(("",port))
server_sock.listen(1)
client_sock,address = server_sock.accept()
print ("Conexion realizada con: ", address)
while True:
recvdata = client_sock.recv(1024)
print ("Informacion recibida: %s" % recvdata)
if (recvdata == "Q"):
print ("Finalizado.")
break
client_sock.close()
server_sock.close()
2 Likes
3.- Bluetooth settings. Paired. Open channel 22.
Before executing the code we must configure the Bluetooth.
sudo apt-get update
sudo apt-get upgrade
sudo apt-get install bluetooth
sudo apt-get install bluez
sudo apt-get install python-bluez
service bluetooth start
service bluetooth status [To quit: Ctrl Z]
sudo bluetoothctl
[bluetooth]# power on
[bluetooth]# agent on
[bluetooth]# discoverable on
[bluetooth]# pairable on
[bluetooth]# scan on
[bluetooth]# pair MAC BLUETOOTH MOBILE
[bluetooth]# paired-devices
bluetooth]# help
[bluetooth]#quit
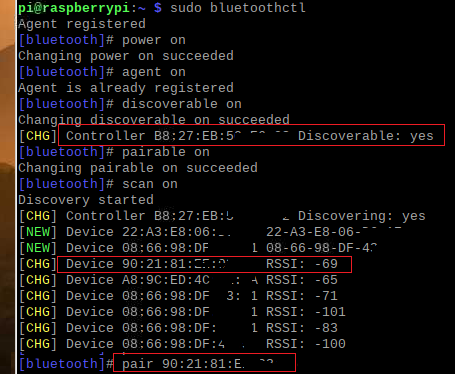
- The communication will be carried out through channel 22. Observe this line in the bluetooth4.py script:
port = 22
sudo sdptool add --channel=22 SP
sudo sdptool browse local
If you get this error: Failed to connect to SDP server on FF:FF:FF:00:00:00:No such file or directory
open, edit this file:
sudo nano /lib/systemd/system/bluetooth.service
and
add --compat in this line:
ExecStart=/usr/lib/bluetooth/bluetoothd --compat
[Ctrl O to save, Ctrl X to quit]
Summary
[Unit]
Description=Bluetooth service
Documentation=man:bluetoothd(8)
ConditionPathIsDirectory=/sys/class/bluetooth
[Service]
Type=dbus
BusName=org.bluez
ExecStart=/usr/lib/bluetooth/bluetoothd --compat
NotifyAccess=main
#WatchdogSec=10
#Restart=on-failure
CapabilityBoundingSet=CAP_NET_ADMIN CAP_NET_BIND_SERVICE
LimitNPROC=1
ProtectHome=true
ProtectSystem=full
[Install]
WantedBy=bluetooth.target
Alias=dbus-org.bluez.service
sudo systemctl daemon-reload
sudo systemctl restart bluetooth
sudo sdptool browse local
channel 22 activated
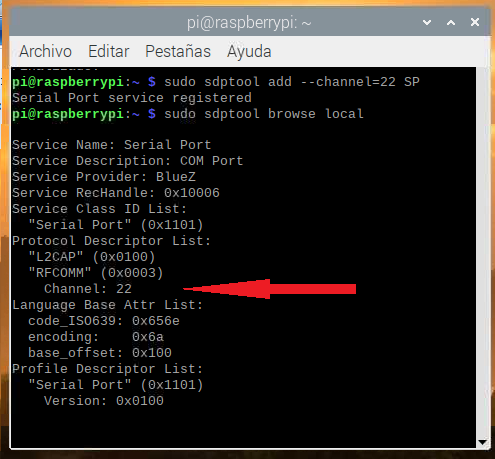
- To run.
- First run the script with Python2
python2 bluetooth4.py
- Then Click the "Inicio - Start" button in the app.
3 Likes
4.- Raspberry Pi sends data to App.
p9B3i_recibir_RaspBerry.aia (2.6 KB)
- Phyton2. Script : bluetooth5.py
import time
import datetime
import bluetooth
server_sock=bluetooth.BluetoothSocket(bluetooth.RFCOMM)
port = 22
server_sock.bind(("",port))
server_sock.listen(1)
client_sock,address = server_sock.accept()
print ("Conexion realizada con: ", address)
# Create class that acts as a countdown
def countdown(h, m, s):
# Calculate the total number of seconds
total_seconds = h * 3600 + m * 60 + s
# While loop that checks if total_seconds reaches zero
# If not zero, decrement total time by one second
while total_seconds > 0:
# Timer represents time left on countdown
timer = datetime.timedelta(seconds = total_seconds)
# Prints the time left on the timer
print(timer)
client_sock.send(str(timer))
client_sock.send("\n")
# Delays the program one second
time.sleep(1)
# Reduces total time by one second
total_seconds -= 1
print("Bzzzt! The countdown is at zero seconds!")
# Inputs for hours, minutes, seconds on timer
h = 0 #input("Enter the time in hours: ")
m = 4 #input("Enter the time in minutes: ")
s = 0 #input("Enter the time in seconds: ")
countdown(int(h), int(m), int(s))
- To run.
- First run the script with Python2
python2 bluetooth5.py
- Then Click the "Inicio - Start" button in the app.
[The countdown script is based on this code:
Create a Timer in Python: Step-by-Step Guide | Udacity]
4 Likes
6.- App sends a number to Raspberry and Raspberry returns double.
p9B3i_enviar_recibirRaspBerry3.aia (3.2 KB)
- Phyton2. Script : bluetooth6.py
import bluetooth
server_sock=bluetooth.BluetoothSocket( bluetooth.RFCOMM )
port = 22
server_sock.bind(("",port))
server_sock.listen(1)
client_sock,address = server_sock.accept()
print ("Conexion realizada con: ", address)
while True:
recvdata = client_sock.recv(1024)
print ("Recibe: %s" % recvdata)
doble = 2 * float(recvdata)
print ("Enviar doble: %s" % doble)
client_sock.send(str(doble))
client_sock.send("\n")
if (recvdata == "0"):
print ("Finalizado.")
break
client_sock.close()
server_sock.close()
- To run.
- First run the script with Python2
python2 bluetooth6.py
- Then Click the "Inicio - Start" button in the app.
4 Likes
Much need information Juan - perhaps it should also be linked in Tutorials and Guides.
Is there a question there? Because you did all the neccesary work to returne double!
You mean the example of sending a value (4.6) and getting the double (9.2), this is an example code, the user can adapt it to their needs, for example, the application requests the temperature from the RPi and it returns it , the application requests the state of a button and RPi returns the state,,.
1 Like
This does not work with Python3 and Raspberry Pi.
Does anyone know how to convert that into Python3 code?