Hello.
I used HC-06 at Arduino to code to send the value to App Inventor.
HC-06 is experiencing problems connecting normally but not delivering values.
Can you tell me the problem by looking at the code?
Arduino Code
#include <SoftwareSerial.h>
#include <HX711.h>
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
const int LOADCELL_DOUT_PIN_1 = 2;
const int LOADCELL_SCK_PIN_1 = 3;
const int LOADCELL_DOUT_PIN_2 = 4;
const int LOADCELL_SCK_PIN_2 = 5;
HX711 scale_1;
HX711 scale_2;
LiquidCrystal_I2C lcd(0x27, 16, 2);
SoftwareSerial hc06(7, 8);
void setup() {
Serial.begin(115200);
Serial.println("HX711 Demo");
lcd.init();
lcd.backlight();
hc06.begin(9600);
Serial.println("Initializing the scales");
scale_1.begin(LOADCELL_DOUT_PIN_1, LOADCELL_SCK_PIN_1);
scale_1.set_scale(-10600.00);
scale_1.tare();
scale_2.begin(LOADCELL_DOUT_PIN_2, LOADCELL_SCK_PIN_2);
scale_2.set_scale(-10600.00);
scale_2.tare();
}
void loop() {
float weight_kg_1 = scale_1.get_units() * 0.453592;
float weight_kg_2 = scale_2.get_units() * 0.453592;
Serial.print("Scale 1 reading:\t");
Serial.println(weight_kg_1, 1);
Serial.print("Scale 2 reading:\t");
Serial.println(weight_kg_2, 1);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Scale 1: ");
lcd.print(weight_kg_1, 1);
lcd.print(" kg");
lcd.setCursor(0, 1);
lcd.print("Scale 2: ");
lcd.print(weight_kg_2, 1);
lcd.print(" kg");
hc06.println(weight_kg_1);
hc06.println(weight_kg_2);
delay(1000);
}
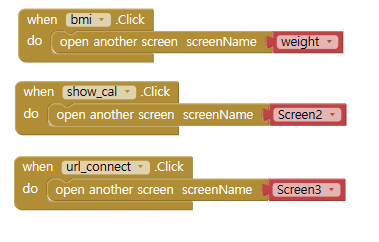