I am seeing contradictions in the ways you are using data types within the sketch and your app.
Here you are defining Notif_var as an unsigned 32 bit integer:
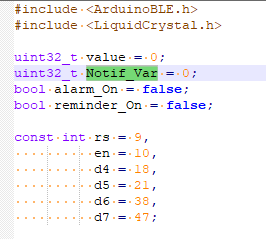
Here you are expecting a compartment number in it:
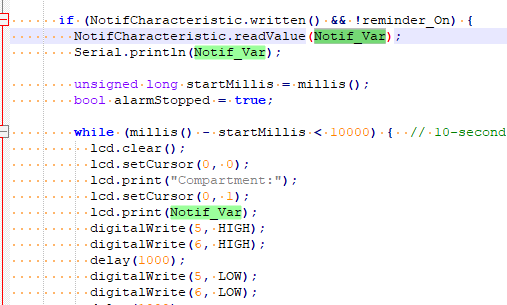
But I don't see any place in your sketch where you are expecting text to arrive from your NotifCharacteristic...
#include <ArduinoBLE.h>
#include <LiquidCrystal.h>
uint32_t value = 0;
uint32_t Notif_Var = 0;
bool alarm_On = false;
bool reminder_On = false;
const int rs = 9,
en = 10,
d4 = 18,
d5 = 21,
d6 = 38,
d7 = 47;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
BLEService PillService("19b10000-e8f2-537e-4f6c-d104768a1214");
// Bluetooth® Low Energy LED Switch Characteristic - custom 128-bit UUID, read and writable by central
BLEUnsignedIntCharacteristic CountCharacteristic("76462190-1639-4f68-9af2-f9f5b3e8f549", BLENotify);
BLEByteCharacteristic NotifCharacteristic("975f4177-fba7-4374-868a-d52603e6b740", BLERead | BLEWrite | BLENotify);
BLEByteCharacteristic FindCharacteristic("875f4177-fba7-4374-868a-d52603e6b741", BLERead | BLEWrite);
void setup() {
Serial.begin(9600);
//while (!Serial); // Uncomment to wait for serial port to connect.
pinMode(5, OUTPUT);
pinMode(6, OUTPUT);
pinMode(7, OUTPUT);
pinMode(8, INPUT);
lcd.begin(16, 2);
// Begin initialization
if (!BLE.begin()) {
Serial.println("Starting Bluetooth® Low Energy failed!");
// Stop if Bluetooth® Low Energy couldn't be initialized.
while (1)
;
}
// Set advertised local name and service UUID:
BLE.setLocalName("Nano-ESP32");
BLE.setAdvertisedService(PillService);
// Add the characteristic to the service
PillService.addCharacteristic(CountCharacteristic);
PillService.addCharacteristic(NotifCharacteristic);
PillService.addCharacteristic(FindCharacteristic);
// Add service
BLE.addService(PillService);
// Set the initial value for the characeristic:
CountCharacteristic.writeValue(value);
NotifCharacteristic.writeValue(Notif_Var);
// start advertising
BLE.advertise();
}
void loop() {
// Listen for Bluetooth® Low Energy peripherals to connect:
BLEDevice central = BLE.central();
lcd.setCursor(0, 0);
lcd.print("Device");
lcd.setCursor(0, 1);
lcd.print("Disconnected");
// If a central is connected to peripheral
if (central) {
Serial.print("Connected to central: ");
// Print the central's MAC address
Serial.println(central.address());
lcd.clear();
// Wait until the central disconnects
while (central.connected()) {
CountCharacteristic.writeValue(value);
value++;
Serial.print("Count value: ");
Serial.println(value);
lcd.setCursor(0, 1);
lcd.print(value);
lcd.setCursor(0, 0);
lcd.print("Device connected");
reminder_On = digitalRead(8);
Serial.println(reminder_On);
if (NotifCharacteristic.written() && !reminder_On) {
NotifCharacteristic.readValue(Notif_Var);
Serial.println(Notif_Var);
unsigned long startMillis = millis();
bool alarmStopped = true;
while (millis() - startMillis < 10000) { // 10-second alarm
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Compartment:");
lcd.setCursor(0, 1);
lcd.print(Notif_Var);
digitalWrite(5, HIGH);
digitalWrite(6, HIGH);
delay(1000);
digitalWrite(5, LOW);
digitalWrite(6, LOW);
delay(1000);
reminder_On = digitalRead(8);
if (reminder_On) {
digitalWrite(5, LOW);
digitalWrite(6, LOW);
alarmStopped = false; // Mark that the alarm was stopped by the button
lcd.clear();
break; // Exit the alarm loop if reminder_On is pressed
}
}
// Write back to the characteristic only after the 10-second timer and if the alarm was not stopped by the button
if (alarmStopped) {
int32_t SMS = 1;
NotifCharacteristic.writeValue(SMS);
Serial.print("Emergency contact: ");
Serial.println(SMS);
lcd.clear();
}
}
if (FindCharacteristic.written()) {
alarm_On = !alarm_On;
Serial.println(alarm_On);
if (alarm_On) {
digitalWrite(6, HIGH);
digitalWrite(7, HIGH);
delay(200);
digitalWrite(6, LOW);
digitalWrite(7, LOW);
delay(200);
digitalWrite(6, HIGH);
digitalWrite(7, HIGH);
delay(200);
digitalWrite(6, LOW);
digitalWrite(7, LOW);
delay(200);
} else {
digitalWrite(6, LOW);
digitalWrite(7, LOW);
}
}
delay(1000);
}
// When the central disconnects, print it out
Serial.print("Disconnected from central: ");
Serial.println(central.address());
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Device");
lcd.setCursor(0, 1);
lcd.print("Disconnected");
digitalWrite(5, LOW);
digitalWrite(6, LOW);
digitalWrite(7, LOW);
}
}
If you are hoping to send a medicine name as text using a Byte stream, you would need blocks to do that conversion. I have some you can use, good only for ASCII text (no accent marks).