Hello!
I have made a control for a remote control airplane that I have as a project, I searched and I didn't find any previously made using WIFI and UDP communication, so with the help of tutorials on this page, chatgpt for the topics that I don't know and a little patience I have managed to create this app, it is a little rudimentary and has errors (for example if you stop pressing the controls it keeps sending the last data and it doesn't return to the data at the midpoint of the controls) but it is good enough to understand what I did and how to make better ones.
If anyone wants more information or thinks they have a better idea than mine, I am open to working together.
Good luck with your projects!
code for the esp32:
#include "WiFi.h"
#include "AsyncUDP.h"
const char *ssid = "ME 410"; // Nombre del AP del ESP32
const char *password = "GIANNA123"; // Contraseña del AP
AsyncUDP udp;
void setup() {
Serial.begin(115200);
WiFi.mode(WIFI_AP); // Modo Access Point
WiFi.softAP(ssid, password); // Configurar AP con SSID y contraseña
Serial.print("IP del ESP32: ");
Serial.println(WiFi.softAPIP()); // Mostrar la IP del ESP32
// Configurar el puerto de escucha UDP
if (udp.listen(1234)) {
Serial.println("Escuchando UDP en el puerto 1234");
// Configurar la recepción de paquetes UDP
udp.onPacket([](AsyncUDPPacket packet) {
// Convertir los datos del paquete en un string
char* tmpStr = (char*) malloc(packet.length() + 1);
memcpy(tmpStr, packet.data(), packet.length());
tmpStr[packet.length()] = '\0'; // Añadir terminación nula
String mensaje = String(tmpStr); // Convertir a String
free(tmpStr); // Liberar la memoria temporal
// Mostrar solo el mensaje en el Monitor Serie
Serial.println("Mensaje recibido: " + mensaje);
// Responder al cliente si es necesario
packet.printf("Recibido: %s", mensaje.c_str());
});
}
}
void loop() {
// No es necesario hacer nada en loop, la recepción es asíncrona
}
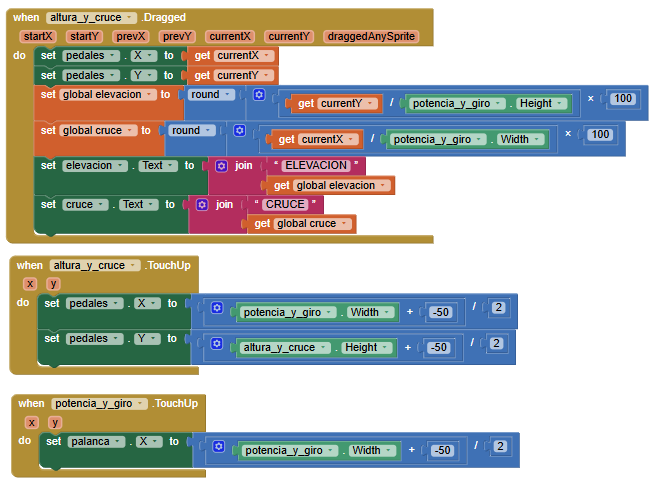
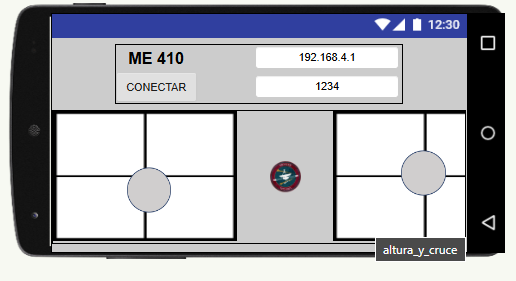