The Gemini extension for AI2 allows you to interact with the Google Gemini-Pro and Gemini-Pro-Vesion models and these models that Bard is based on to generate text and control a stream of text generation.
Features of the Gemini extension for AI2 :
Generate text using the Google Gemini API.
Stream generated text in real time.
Generate text with images.
Encode images to Base64 with the -w0 option.
Handle errors gracefully.
Benefits of using the Gemini extension:
Easy to use: The Gemini extension provides a simple and easy-to-use interface for interacting with the Gemini API.
Powerful: The Gemini API is a powerful tool for generating text, and the Gemini extension makes it easy to use this API in your AI2 apps.
Versatile: The Gemini extension can be used for a variety of tasks, such as generating customer support responses, writing creative content, and translating languages.
Extensible: The Gemini extension is extensible, and new features can be added in the future.
Here are some specific examples of how the Gemini extension can be used:
Create a chatbot that can respond to user queries in a natural and engaging way.
Generate marketing copy for your products or services.
Write blog posts and articles on a variety of topics.
Translate languages in real time.
Create interactive stories and games.
The possibilities are endless!
Blocks
Explanation
Generating Content
To generate content using Gemini, you can use the
GenerateGeminiContent
block. This block takes two arguments:
modelName (String): The name of the Gemini model to use (e.g., "gemini-1.5-flash") check this docs.
apiKey (String): Your Google API key.
contents: A list of dictionaries, where each dictionary represents a content item. Each content item can have ,
So the JSON input forcontents
will be like this[ {"role":"user", "parts":[{ "text": "Write the first line of a story about a magic backpack."}]}, {"role": "model", "parts":[{ "text": "In the bustling city of Meadow brook, lived a young girl named Sophie. She was a bright and curious soul with an imaginative mind."}]}, {"role": "user", "parts":[{ "text": "Can you set it in a quiet village in 1600s France?"}]}, ]
Blocks example:
old Block: for explanationthe following keys:
*role
: A string representing the role of the content item in the conversation.
*parts
: A list of dictionaries, where each dictionary represents a part of the content item. Each part can have the following keys:
*text
: A string representing the text of the part.
- apiKey (String): Your Google API key.
The
GenerateGeminiContent
block will generate content using the specified parameters and return the result in theRespondedToGemini
event.The
RespondedToGemini
event will be triggered with the following parameters:
apiResponse
: The raw API response from Gemini.textParts
: A list of strings representing the generated text parts.role
: The role of the generated content.finishReason
: The reason why the generation was finished.index
: The index of the generated content.safetyRatings
: A list of dictionaries representing the safety ratings of the generated content. Each dictionary will have the following keys:
category
: The category of the safety rating.probability
: The probability of the safety rating.
Streaming Content
To generate content in a stream, you can use the
StreamGenerateGeminiContent
block. This block takes two arguments:
modelName (String): The name of the Gemini model to use (e.g., "gemini-1.5-flash") check this docs.
apiKey (String): Your Google API key.
contents
: A list of dictionaries, where each dictionary represents a content item. Each content item can have the following keys:
role
: A string representing the role of the content item in the conversation.parts
: A list of dictionaries, where each dictionary represents a part of the content item. Each part can have the following keys:
text
: A string representing the text of the part. so the JSON input forcontents
will be like this[ {"role":"user", "parts":[{ "text": "Write the first line of a story about a magic backpack."}]}, {"role": "model", "parts":[{ "text": "In the bustling city of Meadow brook, lived a young girl named Sophie. She was a bright and curious soul with an imaginative mind."}]}, {"role": "user", "parts":[{ "text": "Can you set it in a quiet village in 1600s France?"}]}, ]
Blocks examble
old Block: for explanation
api key
: Your Google Cloud API key.The
StreamGenerateGeminiContent
block will open a stream of content generation using the specified parameters. The generated content will be returned in theGotGeminiStream
event.
The
GotGeminiStream
event will be triggered with the following parameter:
text
: A string representing the generated text.
You can manually stop the stream using the
StopStream
block. TheStoppedStream
event will be triggered when the stream is stopped.
You can also check if the stream is currently running using the
IsStreaming
block.
Generating Content with Images
To generate content using images, you can use the
StreamGenerateGeminiVisionContent
block. This block takes two arguments:
contents
: A list of dictionaries, where each dictionary represents a content item. Each content item can have the following keys:
role
: A string representing the role of the content item in the conversation.parts
: A list of dictionaries, where each dictionary represents a part of the content item. Each part can have the following keys:
text
: A string representing the text of the part.inlineData
: A dictionary representing inline data, such as an image. TheinlineData
dictionary can have the following keys:
mimeType
: The MIME type of the inline data.data
: The base64-encoded data of the inline data.
So the JSON input forcontents
will be like this[ { "text": "Describe what the people are doing in this image:\n" }, { "inlineData": { "mimeType": "image/png", "data": "'$(base64 -w0 image0.jpeg)'" } }, { "text": " " } ] } ]
Blocks example :
old Block: for explanation
api key
: Your Google Cloud API key.
The
StreamGenerateGeminiVisionContent
block will open a stream of content generation using the specified parameters. The generated content will be returned in theGotGeminiStream
event.
StreamGenerateGeminiFileContentFromBase64
This function sends a streaming request to the Google Gemini API to generate content based on the provided files and text.Parameters:
apiKey (String): Your Google API key.
modelName (String): The name of the Gemini model to use (e.g., "gemini-1.5-flash") check this docs.
fileBase64List (YailList): A list of strings containing the Base64 encoded data of the files.
mimeTypeList (YailList): A list of strings containing the MIME types of the files in fileBase64List. The order of MIME types must correspond to the order of files.
additionalText (String): Any additional text to include in the request.
GetGeminiModelNamesThis function retrieves a list of available Gemini model names from the Google Gemini API.
Parameters:
- apiKey (String): Your Google API key.
Events:
- GotGeminiModelNames(List modelNames): This event is triggered when the API request is successful and the list of model names is retrieved. The modelNames parameter contains the list of model names as strings.
- ErrorOccurred(String message, String component): This event is triggered if an error occurs during the API request.
Encoding Images to Base64
The
EncodeImageToBase64
block can be used to encode an image file to Base64 with the-w0
option, which removes all line breaks from the encoded string. This can be useful for sending images to the Gemini API.The
EncodeImageToBase64
block takes one argument:
imagePath
: The path to the image file.The
EncodeImageToBase64
block will return the base64-encoded image as a string.
Error Handling
The
ErrorOccurred
event will be triggered if an error occurs while using the Gemini extension. The event will be triggered with the following parameters:
message
: A string describing the error.component
: The name of the component that caused the error.
Examples
Here is an example of how to use the Gemini extension to generate text:
contents = [{"role": "user", "parts": [{"text": "Hello, Gemini!"}]}] api_key = "YOUR_API_KEY" GenerateGeminiContent(contents, api_key)
Bocks:
Here is an example of how to use the Gemini extension to generate text in a stream:
contents = [{"role": "user", "parts": [{"text": "Hello, Gemini!"}]}] api_key = "YOUR_API_KEY" StreamGenerateGeminiContent(contents, api_key)
Bocks:
Here is an example of how to use the Gemini extension to generate text with images:
contents = [ { "role": "user", "parts": [ {"text": "Here is an image of a cat:"}, {"inlineData": {"mimeType": "image/jpeg", "data": base64_image}} ] } ] api_key = "YOUR_API_KEY"
Bocks:
Here is an example of how to use the Gemini extension to generate text with images in FreeForm Prompt:
you can use this extension to convert the TextBox component to FreeForm layout :
contents = [ { "parts": [ { "text": "Describe what the people are doing in this image:\n" }, { "inlineData": { "mimeType": "image/jpeg", "data": "'$(base64 -w0 image0.jpeg)'" } }, { "text": "\nand what is the relation between this is mage to \n" }, { "inlineData": { "mimeType": "image/webp", "data": "'$(base64 -w0 image1.webp)'" } } ] } ] api_key = "YOUR_API_KEY"
Bocks:
Freeform preview example:
PaLM_2 blocks 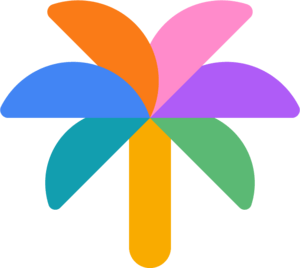
PaLM 2
PaLM 2 is a large language model (LLM) developed by Google that can perform various tasks involving natural language understanding and generation, such as reasoning, coding, mathematics, and multilingual translation. It is an improved version of PaLM, which was released in 2022. PaLM 2 is based on three main innovations: compute-optimal scaling, improved dataset mixture, and updated model architecture and objective. PaLM 2 is also used in other generative AI tools, such as the PaLM API and Bard
Applications that use this extension :
videos preview:
Aix_file:
Check the comparison between PAID and FREE file
PAID_file
Price: $5.99
PayPal payment URL: Purchase Gemini.aix , After payment you will be directed to the download URL so you do not have to contact me to get the extension file however you can contact me in case of any problems
FREE_file
Gemini_Mini.aix (11.6 KB)
Have Inquiries?
For any queries regarding the Gemini extension, feel free to reach out at PM
Note :
*You can try Gemini and get your API key from here