This tutorial is an essay on how to deal with notfound return values from various lookups like
- lookup in pairs
- TinyDB lookup
- lookup in dict
- lookup in FireBase, CloudDB, etc.
Sample code for this article is in ai2.appinventor.mit.edu/?galleryId=6553745952997376 or can downloaded as notfound_confound.aia (275.1 KB)
Block images in this post have been downloaded using AI2’s Download Blocks as Image facility, so they can be dragged directly from this post into your Blocks Editor work space.
The sample data we will use is structured as a lookup table of questions and answer options, indexed by question ID.
Each question is packaged as:
Notice how the lists extend several levels deep here, to collect answer candidates and keep them separate from the question text. Yes, this would be nicer in JSON, but that’s not what we’re about here.
To trigger notfound errors, we will search by a nonexistent question ID, Q9.
We will start with the wrong way to grab data from nonexistent question Q9.
I call it the Leap Before You Look technique …
We see this kind of code produced by optimistic newbies, who don’t think of failure as an option. The error that happens here is
That’s a bit intimidating for newbies, so let’s analyze it…
The operation select list item cannot accept the arguments: , [“not found”], [1]
The two arguments shown here are the inputs to the aforementioned select item from list operation, with the first operand the (supposed) list
and the second operand the index into the (supposed) list.
Unfortunately, "not found’ is not a list, hence the error.
Here’s the cautious way to deal with the possibility of error:
Notice the extra local variable lookupResult I introduced.
We need to put that immediately into quarantine to test it before applying any lookups into it.
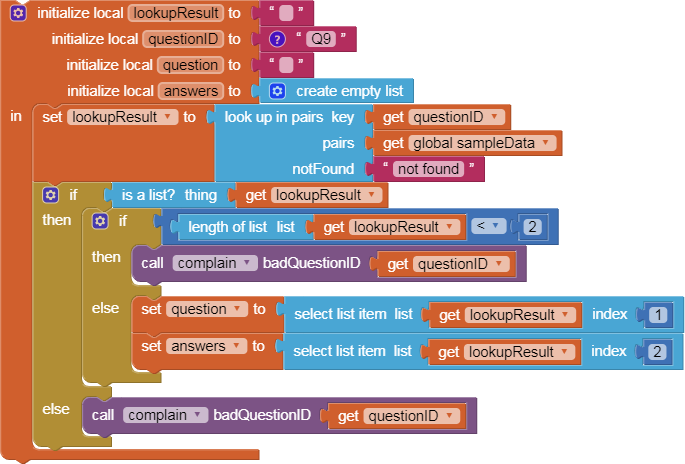
There are at least two ways the lookup result can go wrong, so we test each way, and do not test the second way (does it have a question and answers?) until we have passed the first test (is it a list).
Please note that I did not yet test to see if the list of answers is really a list. I leave that to you as an exercise.
At this point, I introduce a procedure to complain about missing or malformed questions. (My procedure name is too general, I should have named it complainAboutQuestion.)
Here is an extra method to deal with Not Found situations, that replaces pre-testing with a lookup-safe default value return value.
This is a sample return value procedure that creates an empty question with empty answers. It responds to all lookup attempts to extract question text and answers, but with safe values and no error situations. This allows us to use this as a not Found return avlue and avoid having to pre-test before lookup …
I leave it to you to decide which technique works better for you: pre-testing versus customized default structure. Opinions are welcome in this thread.
Summary:
When you code lookups, remember Murphy’s Law:
If anything can go wrong, it will.
Isolate and test your lookup results before diving further into them.