4.- App sends text over WiFi to ESP32, the text is displayed on the VGA monitor.
p250_ESP32_Monitor.aia (2.1 KB)
- Connect the ESP32 as a WiFi client to your local network.
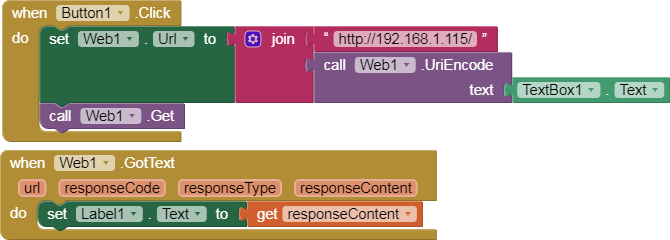
- Code ESP32.
#include <ESP32Lib.h>
#include <Ressources/Font6x8.h>
//pin configuration
const int redPin = 14;
const int greenPin = 19;
const int bluePin = 27;
const int hsyncPin = 0;
const int vsyncPin = 5;
//VGA Device
VGA3Bit vga;
#include <WiFi.h>
const char* ssid = "Nombre_de_tu_red_WiFi";
const char* password = "Contraseña_WiFi";
// Setting Static IP.
IPAddress local_IP(192, 168, 1, 115);
IPAddress gateway(192, 168, 1, 1);
IPAddress subnet(255, 255, 255, 0);
IPAddress primaryDNS(8, 8, 8, 8); //opcional
IPAddress secondaryDNS(8, 8, 4, 4); //opcional
WiFiServer server(80); // Port 80
String retorno = "";
int wait30 = 30000; // time to reconnect when connection is lost.
void setup() {
// vga.init(vga.MODE320x240, redPin, greenPin, bluePin, hsyncPin, vsyncPin);
vga.init(vga.MODE400x300, redPin, greenPin, bluePin, hsyncPin, vsyncPin);
//make the background blue
vga.clear(vga.RGBA(0, 0, 255));
vga.backColor = vga.RGB(0, 0, 255);
//selecting the font
vga.setFont(Font6x8);
vga.setCursor(0, 0);
//displaying the text
vga.println("--- Conectado a VGA ---");
Serial.begin(115200);
// Setting Static IP.
if (!WiFi.config(local_IP, gateway, subnet, primaryDNS, secondaryDNS)) {
Serial.println("Error in configuration.");
}
// Connect WiFi net.
Serial.println();
Serial.print("Connecting with ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("Connected with WiFi.");
// Start Web Server.
server.begin();
Serial.println("Web Server started.");
// This is IP
Serial.print("This is IP to connect to the WebServer: ");
Serial.print("http://");
Serial.println(WiFi.localIP());
}
void loop() {
// If disconnected, try to reconnect every 30 seconds.
if ((WiFi.status() != WL_CONNECTED) && (millis() > wait30)) {
Serial.println("Trying to reconnect WiFi...");
WiFi.disconnect();
WiFi.begin(ssid, password);
wait30 = millis() + 30000;
}
// Check if a client has connected..
WiFiClient client = server.available();
if (!client) {
return;
}
vga.print("New client: ");
vga.println(client.remoteIP());
Serial.print("New client: ");
Serial.println(client.remoteIP());
// Espera hasta que el cliente envíe datos.
// while(!client.available()){ delay(1); }
/////////////////////////////////////////////////////
// Read information sends by client.
String req = client.readStringUntil('\r');
Serial.println(req);
req.replace("+", " "); // Para que los espacios no salgan con +
req.replace(" HTTP/1.1", ""); // Para quitar HTTP/1.1
req.replace("GET /", ""); // Para quitar GET /
vga.println(req.c_str());
retorno = req.c_str();
//////////////////////////////////////////////
// Página WEB. ////////////////////////////
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println(""); // Comillas importantes.
client.println(retorno); // Return.
client.flush();
client.stop();
Serial.println("Client disconnected.");
}