Hello friends,
this is a guide to send information from the application to PC and vice versa via Bluetooth.
There are several ways:
https://groups.google.com/g/mitappinventortest/c/VwgEZu5jH-E/m/Mdo2LMAxBQAJ
https://groups.google.com/g/mitappinventortest/c/KkZbrPI7kb4/m/fTFQkAAaAQAJ
http://www.martyncurrey.com/arduino-and-visual-basic-part-1-receiving-data-from-the-arduino/#more-1700
- In this topic we will see it with Processing.
Processing is a flexible software sketchbook and a language for learning how to code within the context of the visual arts.
Free to download and open source.
For GNU/Linux, Mac OS X, Windows, Android, and ARM.
Processing can also work with Arduino.
https://processing.org/
-
Processing has a size of about 300 MB, it is not necessary to install it, it can work directly, as portable.
1 Like
1.- An example of Processing and Arduino.
-
Arduino + MPU 6050 gyroscope.
-
Moving the gyroscope moves an image with the gyroscope photo on PC screen using Processing, in this case the Arduino is connected to PC by USB cable.
You can find several tutorials on the internet, here I have made one in Spanish:
http://kio4.com/arduino/46Bprocessing.htm
2.- PC with Processing sends data to App by Bluetooth.
We create three Sliders in Processing, when we slide, we send three numbers via Bluetooth to the application.
p46Bi_Processing_BT1.aia (3.9 KB)
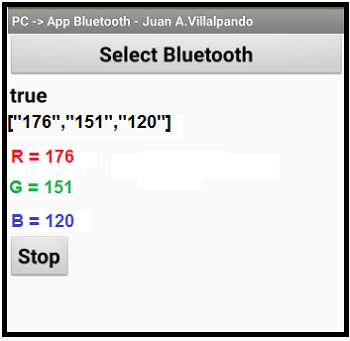
// Juan A. Villalpando
// kio4.com
// http://kio4.com/arduino/46Bprocessing_Bluetooth_AI2.htm
import controlP5.*; // Library create component.
ControlP5 cp5; // cp5 variable library.
import processing.serial.*; // Comunicación Bluetooth.
Serial port; // Port Bluetooth of PC.
int red = 0;
int green = 0;
int blue = 0;
Slider s1, s2, s3;
void setup(){
size(680,380);
background(200,200,200);
println("Availables ports:");
println(Serial.list());
port = new Serial(this, "COM1", 9600); // Port Bluetooth of PC.
cp5 = new ControlP5(this);
PFont p = createFont("Arial",40);
ControlFont font = new ControlFont(p);
cp5.setFont(font);
s1 = cp5.addSlider(" R").setPosition(100,100)
.setRange(0,255)
.setSize(400,40)
.setColorBackground(0xffff0000)
.setColorForeground(0xffaa0000)
.setColorActive(0xffff4400)
.setColorLabel(0xffff0000);
s2 = cp5.addSlider(" G").setPosition(100,150)
.setRange(0,255)
.setSize(400,40)
.setColorBackground(0xffaaff00)
.setColorForeground(0xff009900)
.setColorActive(0xff66ff66)
.setColorLabel(0xff009900);
s3 = cp5.addSlider(" B").setPosition(100,200)
.setRange(0,255)
.setSize(400,40)
.setColorBackground(0xff00aaff)
.setColorForeground(0xff0000ff)
.setColorActive(0xff6666ff)
.setColorLabel(0xff000099);
}
void draw(){
red = int(s1.getValue());
green = int(s2.getValue());
blue = int(s3.getValue());
String Sred = str(red);
String Sgreen = str(green);
String Sblue = str(blue);
port.write(Sred + "," + Sgreen + "," + Sblue + "\n");
}
3.- App sends data to PC with Processing by Bluetooth.
- The application sends three data to Processing via Bluetooth, with three Sliders, for example: 156,176,76#
p46Bi_Processing_BT2.aia (3.8 KB)
- Processing receives three numbers:
// Juan A. Villalpando
// kio4.com
// http://kio4.com/arduino/46Bprocessing_Bluetooth_AI2.htm
import controlP5.*; // Library to create component.
ControlP5 cp5; // cp5 variable of libraru.
import processing.serial.*; // Comunicación Bluetooth.
Serial port; // Port Bluetooth of PC.
String Sred = "";
String Sgreen = "";
String Sblue = "";
char caracter = 0;
String palabra = "";
Textlabel l1, l2, l3;
void setup(){
size(680,380);
background(200,200,200);
println("Puertos disponibles:");
println(Serial.list());
port = new Serial(this, "COM1", 9600); // Port Bluetooth of PC.
cp5 = new ControlP5(this);
PFont p = createFont("Arial",40);
ControlFont font = new ControlFont(p);
cp5.setFont(font);
l1 = cp5.addTextlabel("label1")
.setText("Rojo = ")
.setPosition(100,50)
.setColorValue(0xffff0000)
.setFont(createFont("Arial", 40));
l2 = cp5.addTextlabel("label2")
.setText("Verde = ")
.setPosition(100,100)
.setColorValue(0xff009900)
.setFont(createFont("Arial", 40));
l3 = cp5.addTextlabel("label3")
.setText("Azul = ")
.setPosition(100,150)
.setColorValue(0xff0000ff)
.setFont(createFont("Arial", 40));
}
public void draw() {
while (port.available() > 0) {
caracter = char(port.read());
palabra = palabra + caracter;
if (caracter == '#') {
// Example receives: 123,67,89#
palabra = palabra.substring(0, palabra.length() - 1); // Delete last char #
Sred = getValue(palabra,',',0);
Sgreen = getValue(palabra,',',1);
Sblue = getValue(palabra,',',2);
l1.setText("Rojo = " + Sred);
l2.setText("Verde = " + Sgreen);
l3.setText("Azul = " + Sblue);
background(float(Sred),float(Sgreen),float(Sblue),255);
// port.write("Return: " + palabra + "\n"); // Return data.
palabra = "";
}
}
// background(0);
}
///////////////// Function Split by char ////////////////
String getValue(String data, char separator, int index)
{
int found = 0;
int strIndex[] = {0, -1};
int maxIndex = data.length()-1;
for(int i=0; i<=maxIndex && found<=index; i++){
if(data.charAt(i)==separator || i==maxIndex){
found++;
strIndex[0] = strIndex[1]+1;
strIndex[1] = (i == maxIndex) ? i+1 : i;
}
}
return found>index ? data.substring(strIndex[0], strIndex[1]) : "";
}
4.- Export App to Windows or Linux.
It is possible to create an executable of the application for Windows and Linux.
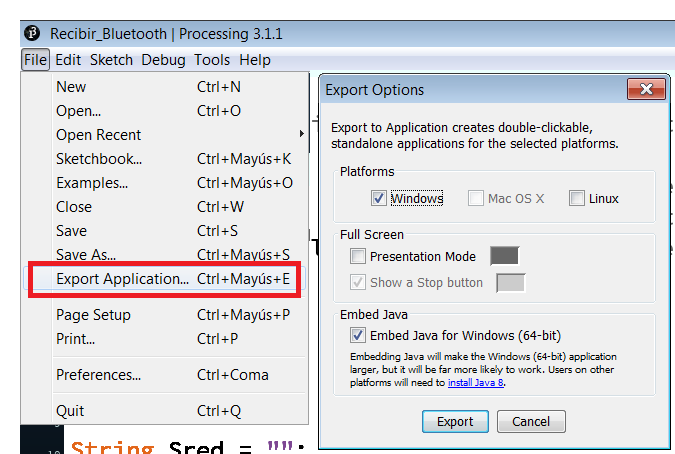
1 Like
Absolutely brilliant Juan!
Looking at Processing, it has a wide range of functionality, very diverse. Such an odd name, even though an accurate name.
1 Like