Hi. I create simple app to change direction of my stepper motor. Everything is working but after 1 or 2 minutes commands sended by bluetooth are lost for example: by 1 or 2 minutes i can run my stepper motor using app properly, after that when i click button once stepper motor is working and once no and after few next seconds i totaly lost bluetooth connection with esp32(i have to run app again and everything works fine again by 1 or 2 minutes). I chcecked app on another device and its the same problem. When i connect my esp32 using serial bluetooth terminal on my device i can run stepper motor all day without any issue.
Any tips for my problem?
Hello @Adrian_Psujek ,
by searching in the forum, you'll find a lot of information about the BT comm's betwen ESP32 and AI2.
For example the following:
For my curiosity: why do you send 1 as a number and 0 as a character ? I believe that in your ESP32 you shall manage the difference ...
When i connect my esp32 using serial bluetooth terminal on my device i can run stepper motor all day without any issue.
This is pretty weird. You know that on the ESP there is a very ennoying watchdog that resets the CPU if not retriggered or if the code remains stuck for some reason, or traps an exception. Have you the possibility to connect your ESP32 to the Serial Monitor of the Arduino IDE and therefore to catch what happens when the BT ceases ? (If the ESP is reset by the watchdog, a series of info is sent automatically by the CPU to the serial monitor)
And why you check twice the same condition with an "else" ?
Hi @uskiara. Thank you for response. I have some more info about my problem. I think the problem is with bluetooth buffering issue when i received information from esp32.
For this moment my app doesnt lost connection but starts to work with big delay after 30 seconds or 1 minute. i tryed to change clock in the app from 10ms to 1000s and also changing const long interval = 1200 in esp32 (when is 1200 aps works without connection lost but with lag and app lost some erceived data)
Below updated code from mit app and from esp32:
#include <AccelStepper.h>
#include <BluetoothSerial.h>
// Ustaw piny dla sterownika DRV8825
#define DIR_PIN 26
#define STEP_PIN 27
// Ustaw piny dla diod LED
int ledPin1 = 13;
int ledPin2 = 12;
int ledPin3 = 14;
// Utwórz obiekt AccelStepper
AccelStepper stepper(AccelStepper::DRIVER, STEP_PIN, DIR_PIN);
// Utwórz obiekt BluetoothSerial
BluetoothSerial SerialBT;
unsigned long previousMillis = 0;
const long interval = 1200;
void setup() {
// Inicjalizuj BluetoothSerial
SerialBT.begin("APLIKATOR"); // Nazwa urządzenia Bluetooth
// Ustaw maksymalną prędkość i przyspieszenie
stepper.setMaxSpeed(1000.0);
stepper.setAcceleration(1000.0);
// Ustaw piny diod LED jako wyjścia
pinMode(ledPin1, OUTPUT);
pinMode(ledPin2, OUTPUT);
pinMode(ledPin3, OUTPUT);
}
void loop() {
// Sprawdź, czy otrzymano dane przez Bluetooth
if (SerialBT.available()) {
char c = SerialBT.read();
// Jeśli otrzymano '1', obróć silnik o 10 kroków w prawo
if (c == '1') {
stepper.move(200);
}
// Jeśli otrzymano '0', obróć silnik o 10 kroków w lewo
else if (c == '0') {
stepper.move(-200);
}
// Jeśli otrzymano '2', zapal diodę 1
else if (c == '2') {
digitalWrite(ledPin1, HIGH);
}
// Jeśli otrzymano '3', zgaś diodę 1
else if (c == '3') {
digitalWrite(ledPin1, LOW);
}
// Jeśli otrzymano '4', zapal diodę 2
else if (c == '4') {
digitalWrite(ledPin2, HIGH);
}
// Jeśli otrzymano '5', zgaś diodę 2
else if (c == '5') {
digitalWrite(ledPin2, LOW);
}
// Jeśli otrzymano '6', zapal diodę 3
else if (c == '6') {
digitalWrite(ledPin3, HIGH);
}
// Jeśli otrzymano '7', zgaś diodę 3
else if (c == '7') {
digitalWrite(ledPin3, LOW);
}
}
// Wywołaj metodę run w każdym obiegu pętli, aby umożliwić ruch silnika
stepper.run();
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval) {
previousMillis = currentMillis;
int sensorValue = analogRead(A0);
float voltage = sensorValue * (5.0 / 4095.0);
voltage = max(0.4f, min(4.5f, voltage));
float pressure_psi = ((voltage - 0.4) / (4.5 - 0.4)) * 100.0;
float pressure_bar = pressure_psi * 0.0689476;
int pressure_whole = int(pressure_bar);
int pressure_decimal = int((pressure_bar - pressure_whole) * 10);
// Wysyłaj wartość ciśnienia przez Bluetooth
SerialBT.print(pressure_whole);
Serial.println();
SerialBT.print(".");
Serial.println();
SerialBT.print(pressure_decimal);
Serial.println();
//SerialBT.println(" bar");
}
}
Also i corrected errors which you saw in my blocks I'm sorry but i'm totaly new in the app's creating so there could be a lot of simple errors in my blocks.
Dear @Adrian_Psujek, never mind, all we've been beginners in something !
Another hint I can tell you is to change the way you receive data: instead of
since you set as receiving terminator the linefeed character [to make it working correctly, remember that the last sending from Arduino shall use a bt.println()] you'd better use the following mode:
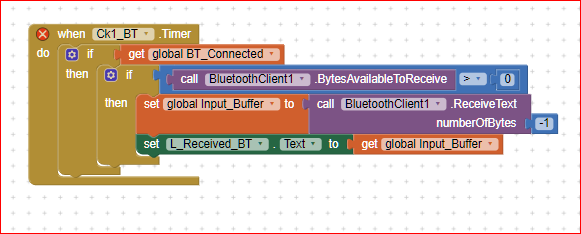
Where the -1 makes the AI2 to wait until the linefeed character is received (and this should avoid characters missing).
As far as the inital delay is concerned, I remember that another guy had the same problem, so if you search the forum with the keywords ESP and Bluetooth, you should find it and see what has been the solution.
One step beyond....
On Arduino you can improve your receiving code as follows (by adding the 'while') :
Second edit:
Change your Arduino sending from this (in which you mixed some serial.println() that are going to the serial monitor not to the serial.BT):
to this:
SerialBT.print(pressure_whole);
SerialBT.print(".");
SerialBT.print(pressure_decimal);
SerialBT.println();
@uskiara
Ok firstly i updated my blocks but i dont know how global_input_bluetooth should look like because i didn't receive any data from esp32.
Nextly i tryed to modify my code adding comends which you suggest. After that i can only connect my app to the esp32 but its disconnected automatly alter while(see picture below from my terminal app)
#include <AccelStepper.h>
#include <BluetoothSerial.h>
// Ustaw piny dla sterownika DRV8825
#define DIR_PIN 26
#define STEP_PIN 27
// Ustaw piny dla diod LED
int ledPin1 = 13;
int ledPin2 = 12;
int ledPin3 = 14;
// Utwórz obiekt AccelStepper
AccelStepper stepper(AccelStepper::DRIVER, STEP_PIN, DIR_PIN);
// Utwórz obiekt BluetoothSerial
BluetoothSerial BT;
char input; // Dodaj zmienną input
unsigned long previousMillis = 0;
const long interval = 500;
void setup() {
// Inicjalizuj BluetoothSerial
BT.begin("APLIKATOR"); // Nazwa urządzenia Bluetooth
// Ustaw maksymalną prędkość i przyspieszenie
stepper.setMaxSpeed(1000.0);
stepper.setAcceleration(1000.0);
// Ustaw piny diod LED jako wyjścia
pinMode(ledPin1, OUTPUT);
pinMode(ledPin2, OUTPUT);
pinMode(ledPin3, OUTPUT);
}
void loop() {
// any character available ?
while (BT.available())
{
// don't miss characters
input = BT.read();
// get the character
switch (input)
{
// do actions related to 1
case '1':
break;
// do actions related to 2
case '2':
break;
// for sake of "switch case" instruction completeness
default:
break;
}
}
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval) {
previousMillis = currentMillis;
int sensorValue = analogRead(A0);
float voltage = sensorValue * (5.0 / 4095.0);
voltage = max(0.4f, min(4.5f, voltage));
float pressure_psi = ((voltage - 0.4) / (4.5 - 0.4)) * 100.0;
float pressure_bar = pressure_psi * 0.0689476;
int pressure_whole = int(pressure_bar);
int pressure_decimal = int((pressure_bar - pressure_whole) * 10);
// Wysyłaj wartość ciśnienia przez Bluetooth
BT.print(pressure_whole);
BT.print(".");
BT.print(pressure_decimal);
BT.println();
//BT.println(" bar");
}
}
So after hours spending on this i have final solution. The problem was in speed of my tablet which i used. It's weird because tablet is pretty fast. When i take my phone(pixel4) and run app everything works perfectly all the time. I trided also on poco f3 and here app also works but sometimes need one or two seconds to think so its not responsible like on pixel 4. Finally app works but i'm afraid when my app will grow up and there will be more data sended from esp32.
Dear @Adrian_Psujek, happy that you solved.
Could it be active in your tablet a battery saver function, that can make the CPU running slow ?
Or search for a hidden app running in the background on the tablet that can damage the responsiveness to any new incoming data frame.
If you feel that the ESP disconnects itself after a while due to a sort of timeout, you can avoid that by implementing a handshake, like a "live" character sent by the app tho the ESP every 5 seconds.
Best wishes.