Probably you have to hard code the functions, like this.
You have these events
@SimpleEvent(description = "Event raised when output returned")
public void GotOutput(final String output) {
EventDispatcher.dispatchEvent(this, "GotOutput", output);
}
@SimpleEvent(description = "Event raised when output result")
public void GotResult(final String output) {
EventDispatcher.dispatchEvent(this, "GotOutput", output);
}
and you do this.
if (something == "GotOutput") {
GotOutput(output);
} else if (something == "GotResult") {
GotResult(result);
}
2 Likes
TIMAI2
22
Yes I could build an if/else block or a switch-case statement to handle things. 
2 Likes
Taifun
23
just pass that name to GotOutput and use it in the event as output parameter
Taifun
2 Likes
Do you want to call a function / method by its name?
1 Like
TIMAI2
25
That is the idea, but to pass it as a parameter ....
2 Likes
Yes. Unfortunately in Java you cannot do
and you cannot do
TextView "myTextViewCreatedJustNow" = new TextView;
and Tim also wants to
as well. It would certainly have challenges.
1 Like
You might try reflection?
2 Likes
Check this, call a function by its name and pass parameters:
TIMAI2
30
I have been thinking about this for sometime
(this is a joke)
I have seen reflection
being used for this on StackOverflow, but the application of the approach was way over my head. 
1 Like
You wont be able to simply pass the method in Java, you could use easy conditions instead.
But still, a similar thing can be done:
package xyz.kumaraswamy.test;
import com.google.appinventor.components.annotations.SimpleEvent;
import com.google.appinventor.components.annotations.SimpleFunction;
import com.google.appinventor.components.runtime.AndroidNonvisibleComponent;
import com.google.appinventor.components.runtime.ComponentContainer;
import com.google.appinventor.components.runtime.EventDispatcher;
public class Test extends AndroidNonvisibleComponent {
public Test(ComponentContainer container) {
super(container.$form());
}
@SimpleEvent
public void AppleFell(int apples) {
EventDispatcher.dispatchEvent(this, "AppleFell", apples);
}
@SimpleEvent
public void OrangeFell(int oranges) {
EventDispatcher.dispatchEvent(this, "OrangeFell", oranges);
}
@SimpleFunction
public void GetApples() throws Exception {
doSomething("...", "AppleFell");
}
@SimpleFunction
public void GetOranges() throws Exception {
doSomething("....", "OrangeFell");
}
private void doSomething(String parameters, String whatEvent) throws Exception {
int counts = parameters.length();
// call the event with the name
// that event has one arg int type
getClass().getMethod(whatEvent,
int.class).invoke(this, counts);
}
}
// second one is event name (any event name in the class)
// but you wont be able to pass method as a paramter
doSomething("....", "OrangeFell");
2 Likes
You might get an idea from dynamic components code it is made upon the principle of reflection 
1 Like
TIMAI2
33
OK, it looks like it will be easier to either:
- Follow Taifun's suggestions in using one event, then select the correct event to run
- Build an if/else or switch/case statement to select the correct event to run
Thanks to all for your ideas and input, it helped me see the wood from the trees 
4 Likes
Taifun
34
@SimpleEvent(description = "Event raised when output returned")
public void GotOutput(final String output, String simpleFunction) {
EventDispatcher.dispatchEvent(this, "GotOutput", output, simpleFunction);
}
@SimpleFunction(description = "set parameters to get output")
public void GetOutput() {
String parameters = "some parameters";
GetFunction(parameters);
}
private void GetFunction(String parameters) {
//do something
//output to event:
GotOutput(output, "mySimpleFunction");
}
Taifun
4 Likes
TIMAI2
36
and with the if/else method, I can use the specific event for the function call
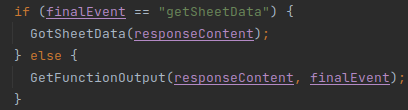
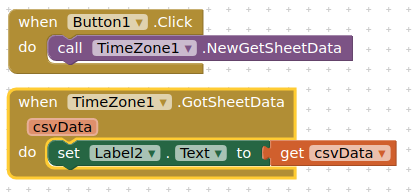
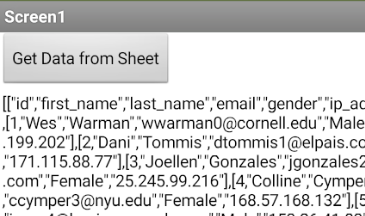
1 Like
TIMAI2
Closed
37
This topic was automatically closed 7 days after the last reply. New replies are no longer allowed.