The formatted sunrise and sunset values seem reasonable... I tried entering the city of New York (about 6h10' time zone). The result is given in European time (Greenweek meridian) from which the 6 o'clock should be subtracted h 10'). With reference to the writings, as already indicated, when the sunset time arrives, the night image must appear and the characters from black must change to white. On the contrary, when it is dawn (sunrise) ... do you have any ideas?
So you really need an app to tell you if the sun is up?
Is it Weather for Cave Dwellers?
For the sake of learning a little logic, here's how to do it.
You will need two variables:
- sunrise_ms
- sunset_ms
After you get sunrise and seconds from the JSON response_content, fill those two variables from 1000 * sunrise and 1000 * sunset (which arrived as seconds.)
Use Clock1.SystemTime as your point of comparison against those two values, now that you are working in milliseconds.
If AND(
- sunrise_ms < Clock1.SystemTime
- Clock1.SystemTime < sunset_ms)
then it is daytime
Else it is night.
The AND block is in the green logical blocks pallette.
Good evening. I have renounced the distinction between day and night (almost useless). Thanks for the information provided. Instead, I'm thinking about how to convert and display dates. For example 2023.08.20
display it, in the European format, as August 20, 2023 and likewise for all the other dates present in the forecasts. Is it possible?
Yes, date conversion can be done most easily with two blocks belonging to the Clock component (from the Sensors Drawer of the Designer):
- Make an Instant
- Format Date Time from an Instant
For your translation pleasure, here is a copy of the Java language datetime format table from SimpleDateFormat (Java Platform SE 7 )
Date and Time Patterns
Date and time formats are specified by date and time pattern strings. Within date and time pattern strings, unquoted letters from 'A'
to 'Z'
and from 'a'
to 'z'
are interpreted as pattern letters representing the components of a date or time string. Text can be quoted using single quotes ('
) to avoid interpretation. "''"
represents a single quote. All other characters are not interpreted; they're simply copied into the output string during formatting or matched against the input string during parsing.
The following pattern letters are defined (all other characters from 'A'
to 'Z'
and from 'a'
to 'z'
are reserved):
Letter Date or Time Component Presentation Examples G
Era designator Text AD
y
Year Year 1996
;96
Y
Week year Year 2009
;09
M
Month in year Month July
;Jul
;07
w
Week in year Number 27
W
Week in month Number 2
D
Day in year Number 189
d
Day in month Number 10
F
Day of week in month Number 2
E
Day name in week Text Tuesday
;Tue
u
Day number of week (1 = Monday, ..., 7 = Sunday) Number 1
a
Am/pm marker Text PM
H
Hour in day (0-23) Number 0
k
Hour in day (1-24) Number 24
K
Hour in am/pm (0-11) Number 0
h
Hour in am/pm (1-12) Number 12
m
Minute in hour Number 30
s
Second in minute Number 55
S
Millisecond Number 978
z
Time zone General time zone Pacific Standard Time
;PST
;GMT-08:00
Z
Time zone RFC 822 time zone -0800
X
Time zone ISO 8601 time zone -08
;-0800
;-08:00
Pattern letters are usually repeated, as their number determines the exact presentation:
-
Text: For formatting, if the number of pattern letters is 4 or more, the full form is used; otherwise a short or abbreviated form is used if available. For parsing, both forms are accepted, independent of the number of pattern letters.
-
Number: For formatting, the number of pattern letters is the minimum number of digits, and shorter numbers are zero-padded to this amount. For parsing, the number of pattern letters is ignored unless it's needed to separate two adjacent fields.
-
Year: If the formatter's
Calendar
is the Gregorian calendar, the following rules are applied.- For formatting, if the number of pattern letters is 2, the year is truncated to 2 digits; otherwise it is interpreted as a number.
- For parsing, if the number of pattern letters is more than 2, the year is interpreted literally, regardless of the number of digits. So using the pattern "MM/dd/yyyy", "01/11/12" parses to Jan 11, 12 A.D.
- For parsing with the abbreviated year pattern ("y" or "yy"),
SimpleDateFormat
must interpret the abbreviated year relative to some century. It does this by adjusting dates to be within 80 years before and 20 years after the time theSimpleDateFormat
instance is created. For example, using a pattern of "MM/dd/yy" and aSimpleDateFormat
instance created on Jan 1, 1997, the string "01/11/12" would be interpreted as Jan 11, 2012 while the string "05/04/64" would be interpreted as May 4, 1964. During parsing, only strings consisting of exactly two digits, as defined byCharacter.isDigit(char)
, will be parsed into the default century. Any other numeric string, such as a one digit string, a three or more digit string, or a two digit string that isn't all digits (for example, "-1"), is interpreted literally. So "01/02/3" or "01/02/003" are parsed, using the same pattern, as Jan 2, 3 AD. Likewise, "01/02/-3" is parsed as Jan 2, 4 BC.Otherwise, calendar system specific forms are applied. For both formatting and parsing, if the number of pattern letters is 4 or more, a calendar specific long form is used. Otherwise, a calendar specific short or abbreviated form is used.
If week year 'Y'
is specified and the calendar doesn't support any week years, the calendar year ('y'
) is used instead. The support of week years can be tested with a call to getCalendar()
.isWeekDateSupported()
.
-
Month: If the number of pattern letters is 3 or more, the month is interpreted as text; otherwise, it is interpreted as a number.
-
General time zone: Time zones are interpreted as text if they have names. For time zones representing a GMT offset value, the following syntax is used:
GMTOffsetTimeZone:
GMT
Sign Hours
:
Minutes Sign: one of
+ -
Hours: Digit Digit Digit Minutes: Digit Digit Digit: one of
0 1 2 3 4 5 6 7 8 9
Hours must be between 0 and 23, and Minutes must be between 00 and 59. The format is locale independent and digits must be taken from the Basic Latin block of the Unicode standard.For parsing, RFC 822 time zones are also accepted.
- RFC 822 time zone: For formatting, the RFC 822 4-digit time zone format is used:
RFC822TimeZone: Sign TwoDigitHours Minutes TwoDigitHours: Digit Digit
TwoDigitHours must be between 00 and 23. Other definitions are as for general time zones.For parsing, general time zones are also accepted.
- ISO 8601 Time zone: The number of pattern letters designates the format for both formatting and parsing as follows:
ISO8601TimeZone: OneLetterISO8601TimeZone TwoLetterISO8601TimeZone ThreeLetterISO8601TimeZone OneLetterISO8601TimeZone: Sign TwoDigitHours
Z
TwoLetterISO8601TimeZone: Sign TwoDigitHours Minutes
Z
ThreeLetterISO8601TimeZone: Sign TwoDigitHours
:
Minutes
Z
Other definitions are as for general time zones or RFC 822 time zones.For formatting, if the offset value from GMT is 0, "Z"
is produced. If the number of pattern letters is 1, any fraction of an hour is ignored. For example, if the pattern is "X"
and the time zone is "GMT+05:30"
, "+05"
is produced.For parsing, "Z"
is parsed as the UTC time zone designator. General time zones are not accepted.If the number of pattern letters is 4 or more, IllegalArgumentException
is thrown when constructing a SimpleDateFormat
or applying a pattern.
SimpleDateFormat
also supports localized date and time pattern strings. In these strings, the pattern letters described above may be replaced with other, locale dependent, pattern letters. SimpleDateFormat
does not deal with the localization of text other than the pattern letters; that's up to the client of the class.
Examples
The following examples show how date and time patterns are interpreted in the U.S. locale. The given date and time are 2001-07-04 12:08:56 local time in the U.S. Pacific Time time zone.
Date and Time Pattern Result "yyyy.MM.dd G 'at' HH:mm:ss z"
2001.07.04 AD at 12:08:56 PDT
"EEE, MMM d, ''yy"
Wed, Jul 4, '01
"h:mm a"
12:08 PM
"hh 'o''clock' a, zzzz"
12 o'clock PM, Pacific Daylight Time
"K:mm a, z"
0:08 PM, PDT
"yyyyy.MMMMM.dd GGG hh:mm aaa"
02001.July.04 AD 12:08 PM
"EEE, d MMM yyyy HH:mm:ss Z"
Wed, 4 Jul 2001 12:08:56 -0700
"yyMMddHHmmssZ"
010704120856-0700
"yyyy-MM-dd'T'HH:mm:ss.SSSZ"
2001-07-04T12:08:56.235-0700
"yyyy-MM-dd'T'HH:mm:ss.SSSXXX"
2001-07-04T12:08:56.235-07:00
"YYYY-'W'ww-u"
Good morning Antonio. I'm proceeding to enter the air quality. It doesn't work for me... I can't get "co","no","no2" out of the json..... where did I go wrong? Thank you
Your path to the co is wrong.
It should be a list with three items
- 'list'
- 'components'
- 'co'
You gave it a list with two items.
It's time to learn how to work with lists.
Look for the new tutorial in the tutorials section, by Saj
error
Unable to parse key as integer: components
Oops, I missed that [ after list.
It should be 4 items:
- list
- 1
- components
- co
Good morning Antonio, now it's fine ... I'm learning a lot ... I've been dealing with appinventor 2 for just over a month ... thanks
Good evening. I'm trying to convert dates, like 2023-09-02 to 02-09-2023 but in my appinventor 2 I'm missing the "datepicker1" and "timepiker1". Do I need to update the app? Or these components no longer appear? Who replaces them? Thank you
If you already have the dates in that form, you can use the text SEGMENT block to extract parts and JOIN blocks to reassemble them as you like.
Good evening Anthony. I recently restarted my app. I find it difficult to convert the date, for example
2023-09-02 to 02-09-2023 or
2023-09-02 to 02-Sep-2023
Can you correct or change the block I created? Thank you
While we wait for Antonio, here is a draggable value function you can use.
Antonio
Antonio
Wherefore art thou, Antonio
(Antonio and Juliet, by William Shakespeare)
Grazie
This topic was automatically closed 7 days after the last reply. New replies are no longer allowed.
Good evening Antonio. I can't tell when one time is less or more than another. In the past you gave me the explanation in the image. Can you correct the block I created since it gives me the error you see in the image? Thank you
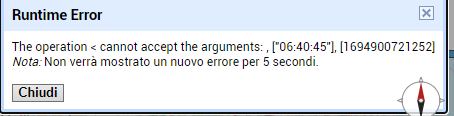
(Canned Reply ABG - Public Only)
I do not provide private support.
You will get more informed and quicker worldwide support by asking in a new public thread.
Making this thread public.
You did not create the two variables I requested to hold the milliseconds for the comparison.